Angular - HTTP Request
Introduction
An application communicates with the backend by an Http Request. This request goes to the server which access database, files or other resources necessaries on the server-side.
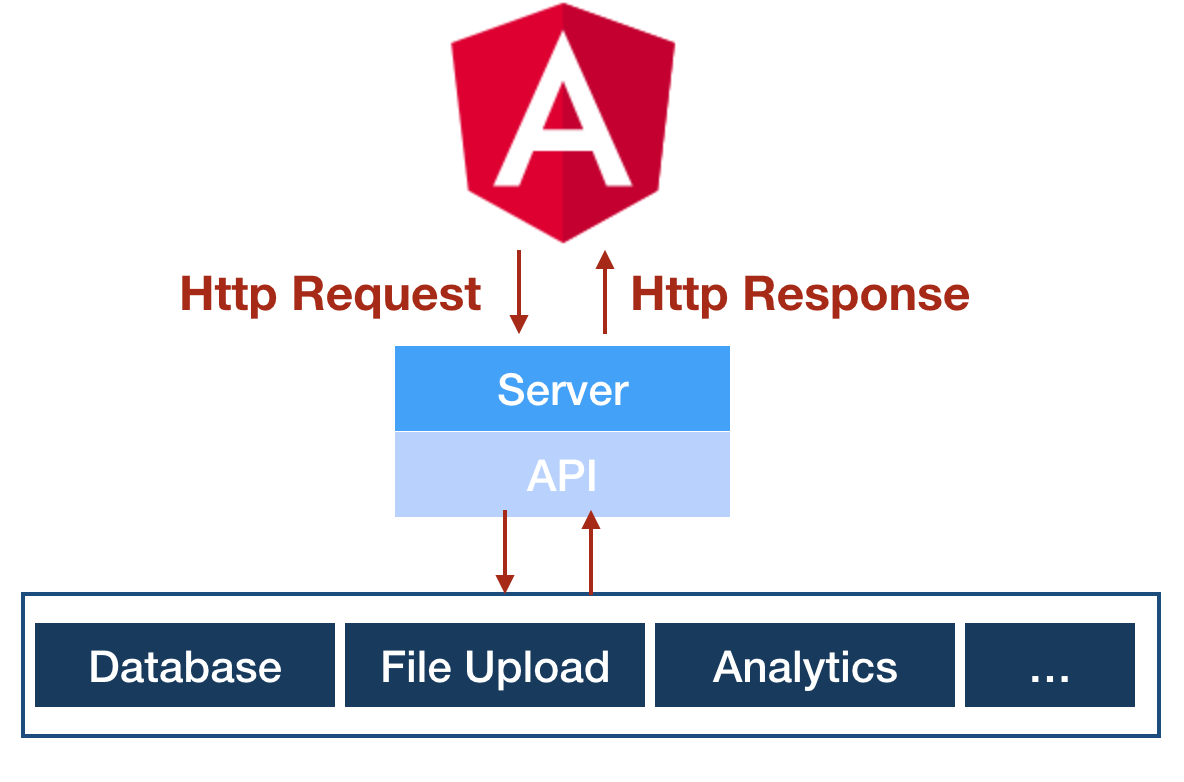
The application using Angular does the HTTP request through the HttpClient which uses XMLHttpRequest. The HttpClient is inside the common/http package.
To use this API you should import HttpClientModule in AppModule. To use, you need to inject that.
// 1. Declaration import { HttpClient } from '@angular/common/http'; @NgModule({ imports: [ ... HttpClientModule, ... // 2. Using import { HttpClient } from '@angular/common/http'; @Injectable() export class YourService { constructor(private http: HttpClient) { } }
Access the backend
The Http Request has four parts:
- Http Verb: POST, GET, PUT, DELETE
- URL (API Endpoint): /clients/1
- Headers (Metadata): {Content-Type: application/json}
- Body: { title: "My new Post"}
A simple example to access the backend is to rescue data from the server using the verb GET. The return of that request is an observable that can be used in the subscribe call. The complete example you can see here.
this.http.get('http://domain.com/opendata/AIn01') .subscribe (resp => console.log(resp));
The return can be mapped to a local model defined as an interface. However, if you need the complete response you can cast to the HttpResponse.
// Your model export interface YourModel { name: string; email: string; } // Casting to your model this.http.get<YourModel>('http://domain.com/opendata/AIn01') .subscribe (resp => console.log(resp.name + ":"+ resp.email)); // Complete Response yourMethod(): Observable<HttpResponse<YourModel>> { return this.http.get<YourModel>( 'http://domain.com/opendata/AIn01', { observe: 'response' }); }
In case to send data to the server, you need to change the verb. For example, if you will send data, you need to use POST or PUT verb.
this.http.post<YourModel>( 'http://domain.com/opendata/AIn01',yourObject)
You can follow the same idea to DELETE.
With this API also is possible to handle errors, using operators to manage the data, customize the header.