Angular Router
Routing is the concept you will use when your app needs to navigate. The difference between using an Angular router and a common link is the performance.
One option to start to work with the router in your project is to add a constant in the AppModule class and declare that in the imports attribute.
import { Routes, RouterModule } from '@angular/router'; ... const appRoutes: Routes = [ { path: '', component: HomeComponent }, { path: '**', component: PageNotFoundComponent } { path: 'home', component: HomeComponent }, { path: 'contactus', component: ContactusComponent } ... @NgModule({ ... imports: [ ... RouterModule.forRoot(appRoutes) ] ]; ... export class AppModule { }
The ‘**’ is the wildcard, used to redirect any page that is not mapped.
Pay attention that the paths don't have the slash (/), representing the absolute path. More detail to use relative paths you can see here.
Another option to the route is, in the creating moment, where you say " to the question "Would you like to add Angular routing?". It will create the app-routing.module.ts file and add in app.module.ts. The constant Routes is where you will put all of the addresses used by your app to navigate.
If you say "no", yet is possible to add the router manually in another moment of your development.
A Simple Example
To test the use of routing let's create components to navigate. The first example (based on tutorialspoint) I created the component home and contactus. After that, I added the pair path and component into Routes constant, then export this const, and finally, add this into app.module.ts.
To test that, update the app.component.html and click on the links. You will see the result.
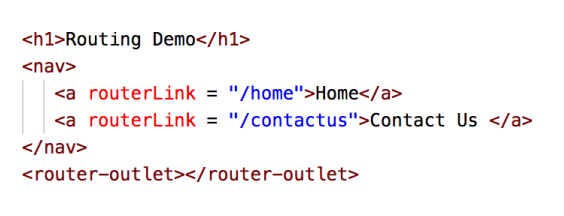
The RouterOutlet
is a directive from the router library that is used like a component.
The routerLink is responsible for the navigation. Also, you can retrieve the router state using the tree created at the end of the navigation lifecycle.
Also, you can add another attribute to indicate if the link is active or not. It’s possible just use class=’active’ from bootstrap or use a second solution:
<a routerLinkActive= "active" routerLink="/home">Home</a>
<a routerLinkActive= "active" routerLink="/contactus">Contactus</a>
If you need to use parameter to route, you can add the parameter in the router map or by link.
// router map { path: 'contactu/:id', component: ContactusComponent } // By link <a [routerLink]="['contactus, contact.id]">
Conclusion
The router is not a big deal to start to create. However, it is an important part of a project and should have a good plan to not be a mess.
A simple example you can see in my gitHub.