Maven - an overview!
The process of assembling a complete environment for development can be necessary several steps until you start to code. This process is easier if you use some tools that will help you with this steps. The tools can be Ant, Maven and Gradle.
Ant
Years ago, Ant was the most popular automation tool, excels at build process. It is used for building Java applications. Additionally, Ant can be used for building non-Java applications. Ant build files are called build.xml.
One big problem with Ant is the complexities that XML build files can be, making this hard to maintain. It happens because Ant does not impose any conventions.
Ant is procedural, then, work with Ant can be verbose because you have to tell exactly what to do, where your source is, etc. And Ant doesn’t have a lifecycle. Beside, Ant comes with limitations due to not having built-in support for dependency management.
Maven
Maven is a project management tool that encompasses a Project Object Model, a set of standards, a project lifecycle, a dependency management system, and logic for executing plugin goals at defined phases in a lifecycle. When you use Maven, you describe your project using a well-defined Project Object Model, Maven can then apply cross-cutting logic from a set of shared (or custom) plugins.
Maven can be considered a build tool or a project management tool. In addition to providing build capabilities (preprocessing, compilation, packaging, testing, and distribution), Maven can also run reports and generate a website. Primarily used with Java-based projects but that can also be used to manage projects in other programming languages like C# and Ruby.
Apache Maven has conventions and provides the available targets (goals) that can be invoked. Then, Maven makes a project easy to build and provides a uniform build process (maven project can be shared by all the maven projects). Moreover, Maven introduced the ability to download dependencies over the network (Ant got this with Ivy).
With maven, the project structure has been standardized and there is no need to define each of the phases in the build process manually, as it was necessary with Ant. Also, Maven has the idea of default behaviours for projects, and the default source code is assumed to be in ${basedir}/src/main/java and resources are assumed to be in ${basedir}/src/main/resources. Tests are assumed to be in ${basedir}/src/test, and a project is assumed to produce a JAR (Java ARchive) file. Maven assumes that you want to compile bytecode to ${basedir}/target/classes and then create a distributable JAR file in ${basedir}/target. It can be customized.
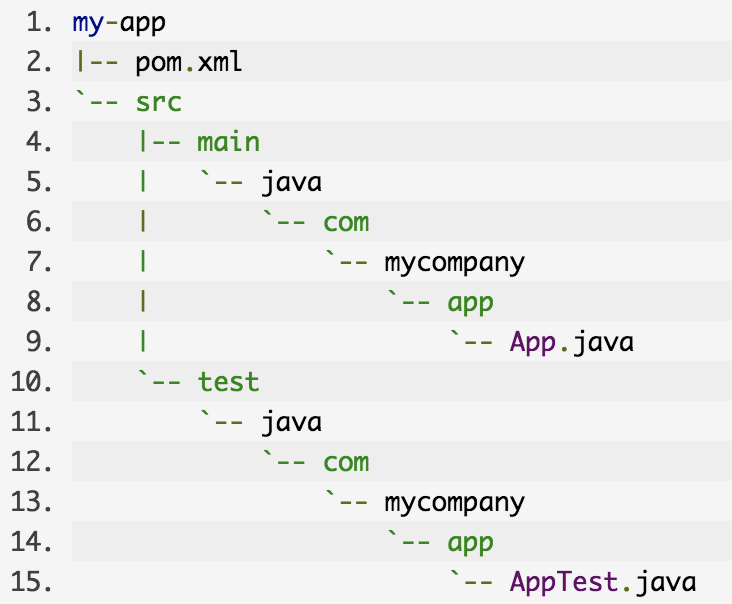
The fundamental unit in Maven is the Project Object Model (POM), an XML file that includes information about the software project, configuration details, dependencies and the build order. A POM can be extended by other POM.
POM | Plugin |
---|---|
![]() |
![]() |
The core of the Maven is very simple. Maven delegate most of the responsibilities to a set of Maven plugins and the plugins are retrieved from the Maven repository. The plug-ins provide a set of goals that can be executed. Plug-ins are configured in the POM file, where some basic plug-ins are included by default.
Examples of a plugin are the Maven Jar plugin that contains goals for creating JAR files, the Compiler plugin that contains goals for compiling the source code and unit tests, or the Surefire plugin that contains goals for executing unit tests and generating reports.
A goal is a “unit of work” in Maven and may be executed as a standalone goal or along with other goals as part of a larger build.
Summary of Main features:
Maven Lifecycle
The build lifecycle is an ordered sequence of phases involved in building a project. Plugin goals can be attached to a lifecycle phase. As Maven moves through the phases in a lifecycle, it will execute the goals attached to each particular phase. Each phase corresponds to zero or more goals.
There are three pre-defined lifecycles: default (handles your project deployment - build and deploy), clean (handles project cleaning - clean the project, remove build artifacts from working directory) and site (handles the creation of your project’s site documentation).
The default lifecycle, for example, has this phase and they are executed sequentially.

To the clean lifecycle, the goal is clean and it has 3 phases:
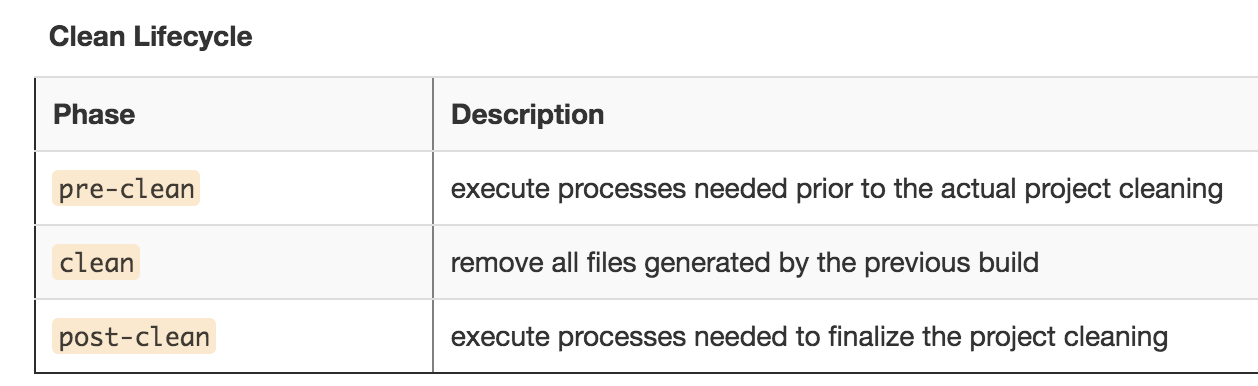
To the site lifecycle you have 4 phases:
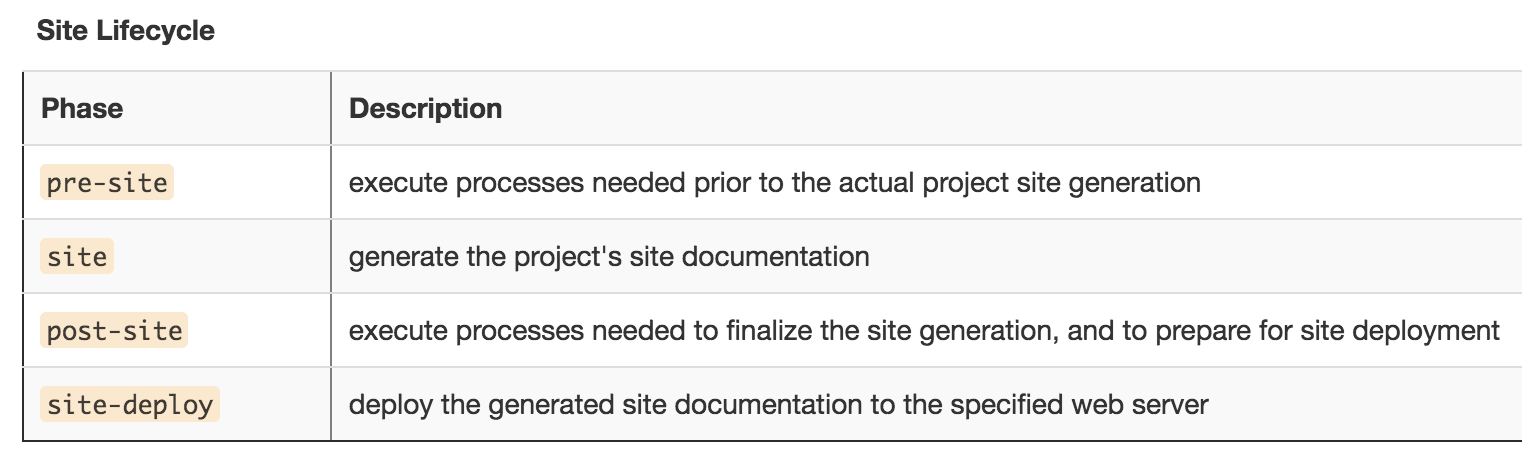
There are intermediate phases that are not usually directly called from the command line. In the default lifecycle, e.g, between validate and compile phases, exist initialize, generate-sources, process-sources, generate-resources and process-resources. Between compile and test you have: process class, generate test source, process test source, generate test resources, process test resources, test compile, process test classes. Between test and package there is prepare package. And between package and verify there are: pre-integration test, integration test, post integration test.
If you use $mvn install all phases before that will be executed. If you use $mvn clean deploy, the build will be clean (delete the build directory - ${basedir}/target) and the artifacts will be deployed to a remote repository.
It's possible to add new goals in a phase to be executed. It 's configured in the pom.
Archetype
Archetype is a Maven project templating toolkit. An archetype is defined as an original pattern or model from which all other things of the same kind are made
The basic archetype is the quickstart, and it can be used:
$mvn archetype:generate -DartifactId=produtos -DgroupId=br.alura.maven -DinteractiveMode=false -DarchetypeArtifactId=maven-archetype-quickstart
- The -Dname=value pairs are arguments that are passed to the goal and take the form of -D properties
- archetype:generate is called a Maven goal -> describe a unit of work to be completed in a build.
Maven Repositories
Maven ships with the bare minimum and fetches from a remote repository when it needs to. The first run will download a number of files from a remote Maven repository. The artifacts and plugins are downloaded to a local repository (where the project artifacts are stored). Once Maven has downloaded an artifact from the remote repository, it never needs to download that artifact again. Maven uses the local repository to share dependencies. The maven search by dependencies at least once a day, but can be configured.
USERNAME.m2\repository
Nexus is a manages software “artifacts” to share artifacts within an organization.
Maven Dependencies
A project can have more than one [dependency](http://www.mvnrepository.com) and it might contain dependencies that depend on other artifacts. It is transitive dependencies. When it happens, the maven is responsible to download this dependency. Maven will also take care of working out conflicts between dependencies, and provides you with the ability to customize the default behaviour and exclude certain transitive dependencies.
Maven Scopes
- Test: The dependencies will be available on the classpath only during the test compilation and test execution
- Compile: It is the default [scope](https://www.baeldung.com/maven-dependency-scopes). Dependencies are available on the classpath of the project in all build tasks and they’re propagated to the dependent projects.
- Provided: dependencies that should be provided at runtime by JDK or a container. The dependency is needed for compilation, but should not be bundled with the output of a build.
- Runtime: the dependencies will be present in runtime and test classpath, but not needed for compilation of the project code.
Simple example
- Download maven
- Add maven in the path to run in every directory
- Create the first project using the quickstart archetype:
- $mvn archetype:generate -DartifactId=produtos -DgroupId=br.alura.maven -DinteractiveMode=false -DarchetypeArtifactId=maven-archetype-quickstart
- $workspace/projectnames$ mvn compile
- $workspace/projectnames$ mvn test
- offline execution
- $workspace/projectnames$ mvn clean
- $workspace/projectnames$ mvn surefire-report:report
- This plugin is not a standard plugin
- $workspace/projectnames$ mvn package
- $workspace/projectnames/target$ java -cp projectname-1.0-SNAPSHOT.jar package.App
- Run the application
SNAPSHOT
This suffix is used by maven to define the maven's behaviour. It identify to maven that it's regarding to a development version and it's not a stable version, then maven will check for newer versions.
Tip
A good course you can see in the Udemy - Apache Maven: Beginner to Guru.
Gradle
Gradle is an open-source build automation tool focused on flexibility and performance.
We can say that Gradle will substitute Maven because it does what maven do and have more performance. It deserves a complete post about this. So, in another moment I will be back to this topic. For now, look a post that compares maven and Gradle here.
Summary
https://youtu.be/nt1n4UyXQXo