Java Net
This post give a overview regarding the javaNet. Most part of this studies used the Book Java Net Programming 4th. You can see some codes used in this post here.
Access the resource
Some functionalities involve access to external resources. Maybe your solution can be using simple access by address. This post wants to explore a little bit about this subject.
URLConnection
The URL identifies a resource and provides the network address of it. The location includes the protocol (e.g. file, ftp, http, https), the hostname (or IP) and the path:
- sintax: protocol://userInfo@host:port/path?query #fragment
- authority: userInfo + host + port
Bellow, an example where you open a connection with google and can access the content type.
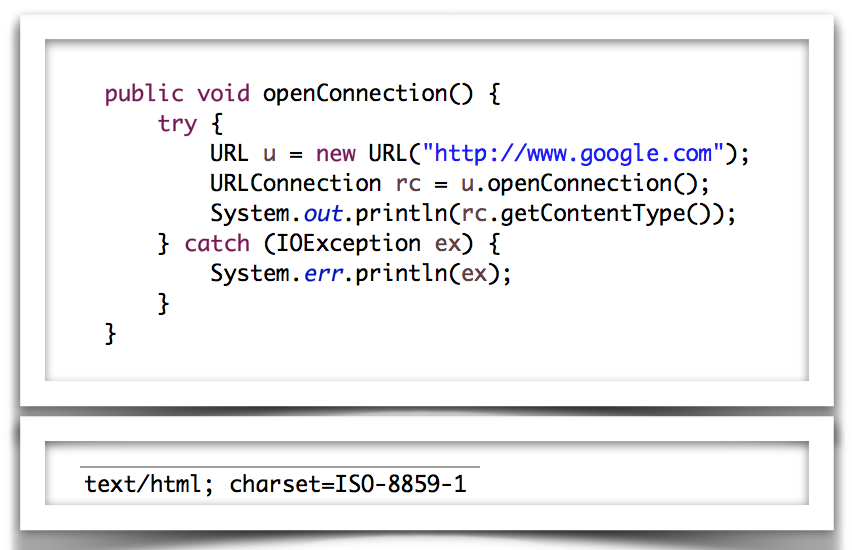
The openConnection() method opens a socket to the specified URL (which represents a resource) and returns a URLConnection object. This object helps to control the interaction with a server. With this object, you will have access, for example, to the content, the header, the host, the path and the port. Therefor, it can send data back.
The URLConnection class is an abstract class from java.net and has as major subclass the HttpURLConnection, from sun.net, specific to work with http protocol.
InetAdress
It is another way to access the resource. This class is Java’s high-level representation of an IP address. Using this is possible to have name, host and address, but not content.
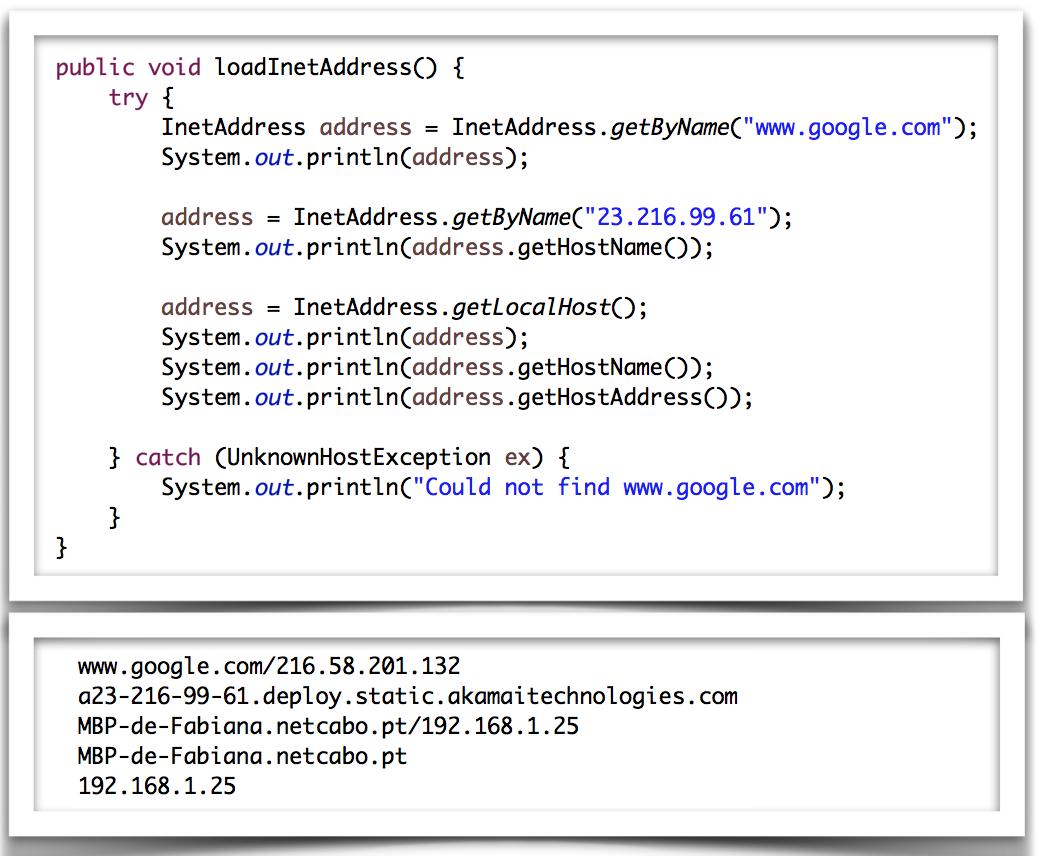
Read the content
However, usually you need more than that. Read and write, for example.
The general steps to use the URLConnection, which you may or may not use are [1]
- Construct a URL object.
- Invoke the URL object’s openConnection() method to retrieve a URLConnection object for that URL.
- Configure the URLConnection
- Read the header fields.
- Get an input stream and read data.
- Get an output stream and write data.
- Close the connection
To access the content, you will have two ways to do it. One way is access it by getContent method which return a complete object to be cast to the type defined by contentType in header. The second is by openStream method, which envolve InputStream. The streams are more useful and dynamically because it move bytes from origin and a target. Both implicitly create a connection to the remote URL object. In this case, the http method used is the GET.
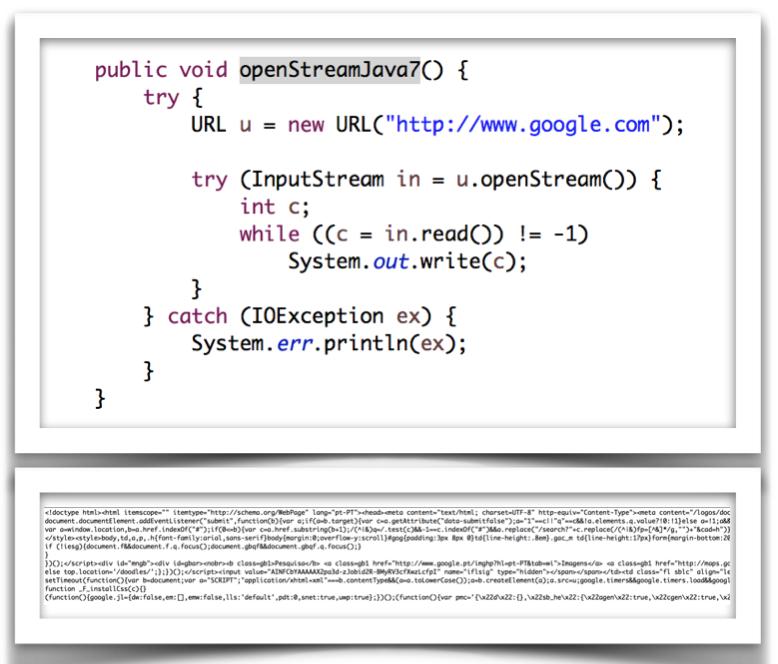
Other cases, you need save this data. Here are some examples how you can do this: [2],[3].
Furthermore, you can use buffer to improve the performance. It make the future reads much faster. In this case, there are classes to read and write as for example BufferedInputStream and BufferedOutputStream.
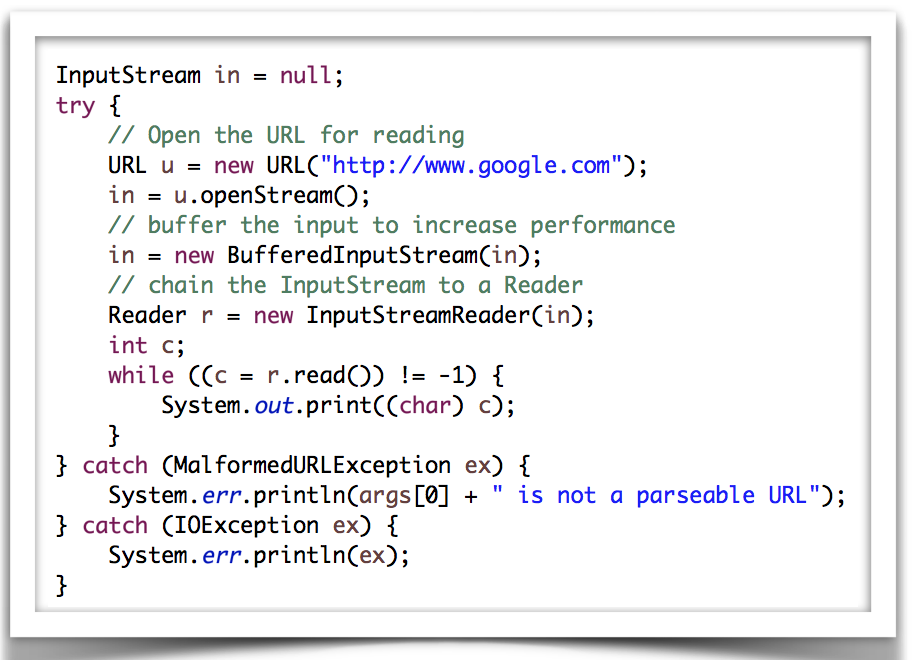
Write the content
If you want submit data to the server, you are doing a POST or a PUT, for example. In these cases, you will start the process to write the data.
The default method used by URLConnection is the GET. Then, the intention to write must be explicitly using the method setDoOutput to config that. The getOutputStream is used to write the query string.
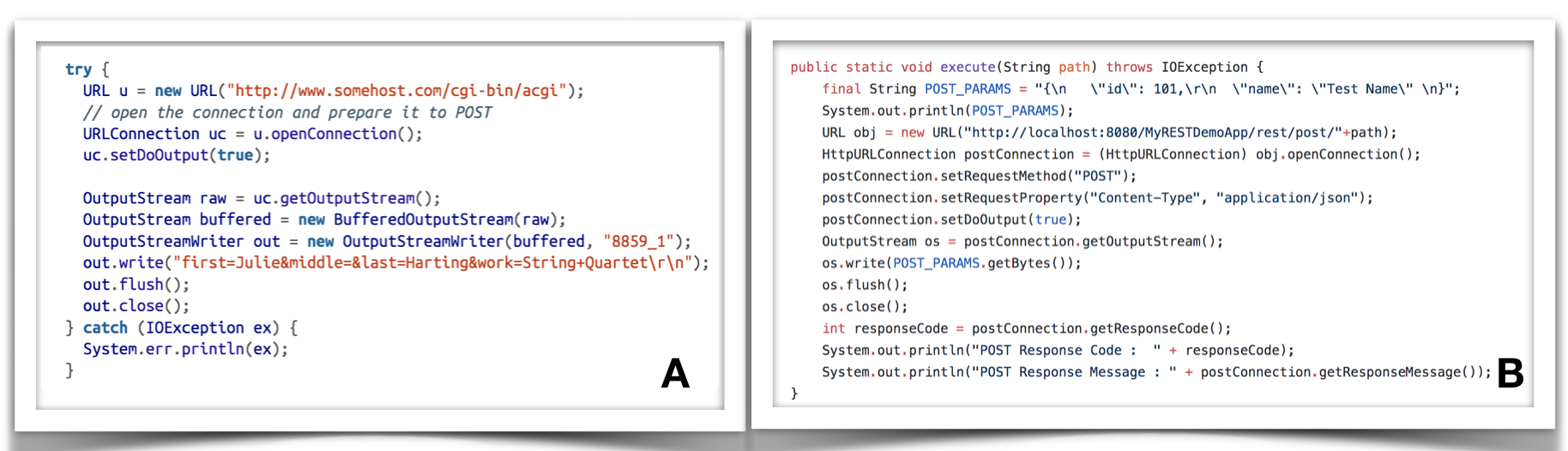
The code A is a simple example using buffer obtained from [1]. The second code [4] , B, is an example where is explicitly defined the method and the content type. This last example was used in my last post regarding Rest.
Other examples of code to write on server:
PS: For sure, also you can use other methods: HEAD, DELETE, OPTIONS and TRACE.
Important classes of the streams
- InputStreamReader/OutputStreamWriter: subclass of Reader/Writer. The reader translates the bytes into Unicode characters using the specified encoding. The writer receives the unicode characters and translates into bytes using the specified encoding and writes the bytes onto an underlying output stream.
- BufferedOutputStream / BufferedInputStream: storage/read data to/from a buffer
- BufferedReader/ BufferedWriter Similar the classes before. However, the before classes use an internal array of bytes and this two use use an internal array of chars.
- PrintStream: To print the data
- The DataInputStream / DataOutputStream: reading/write Java’s primitive data types and strings in a binary format.
Proxy
Sometimes, you are working in a project where the application is behind a proxy. You should config this before access the URL.
Proxy proxy = new Proxy(Proxy.Type.HTTP, new InetSocketAddress(“127.0.0.1”, 8080)); conn = new URL(urlString).openConnection(proxy);
Good examples using proxy you can find here: [5], [6], [7].
Related Subjects
Cache
- Oracle: URLConnection caching API
- codota: How to usesetUseCachesmethodinjava.net.URLConnection
- Java Code Simple
Reference
- [1] Java Net Programming 4th
- [1.1] PDF - Java Net Programming 4th
- [2] Java Download File from URL
- [3] Java HttpURLConnection to download file from an HTTP URL
- [4] Rest - Providers and Interceptors
- [5] Oracle - Java Networking and Proxies
- [6] Connecting Through Proxy Servers in Core Java
- [7] How to useProxyinjava.net
- [8] Do a Simple HTTP Request in Java
- [9] Java HttpURLConnection Example – Java HTTP Request GET, POST
- [10] HttpURLConnection
- Java Sockets: Criando comunicações em Java
- Java Socket: Entendendo a classe Socket e a ServerSocket em detalhes