XML-Based Web Service
A web service is a functionality accessed over the network by other application in a different format (XML, JSON, etc). The web services are reusable and each service should be independent of each other.
Web services are client and server applications that communicate over the World Wide Web’s (WWW) HyperText Transfer Protocol (HTTP). As described by the World Wide Web Consortium (W3C), web services provide a standard means of interoperating between software applications running on a variety of platforms and frameworks. [1]
The two types of web services are:
- SOAP: it is a standard protocol to create web services. it's an XML standard based where the communication can happen by the use of different protocol such as HTTP or FTP. The messages are expressed only by the XML format. The services description are written in the Web Services Description Language (WSDL) which can describe operations, bindings and the location of the web service. It uses well-defined protocols and cannot use REST services. To security, it uses WS-Security. The API is JAX-WS (JSR 224).
- REST: it is an architectural style to build web services. it's the simplest way to develop a web service where the service can be identified by the URI. The data can be expressed by different formats (XML, JSON, etc) and the protocol to use is HTTP. It does not need a WSDL, one reason to REST requires less bandwidth. The REST is stateless and cachable. The security is from the underlying transport layer. Also, the REST can use SOAP services and the API used is JAX-RS (JSR 311).
The API’s are part of Java EE API’s.
You should use WS when QoS is required and when security and reliability are important. REST should be chosen if scalability and architectural simplicity are important [2]. A comparative you can see here.
This post will talk about SOAP. The REST will be explored in another post.
Components
The XML-based Web Service has three components that you need to know.
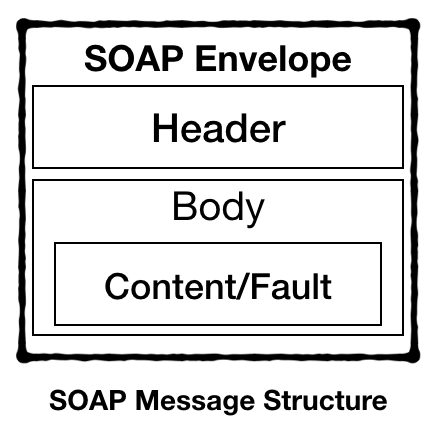
- SOAP (Simple Object Access Protocol ): it is an XML-based protocol and message formats using XML. It is a document that contains an envelope (identify the document), header, body (request/response) and fault (error/status) elements.
- WSDL(Web Services Definition Language): it is a standard web service description (location, methods).
- UDDI (Universal Description, Discovery and Integration): it's a standard to a dynamic discovering of web service (describing, publish and find).
SOAP | WSDL |
---|---|
![]() |
![]() |
Architecture
Roles
- Provider: implements and makes the service available.
- Requestor: the consumer of the service by an XML request.
- Registry: a central place where the service is published.
Sometimes a service can be a provider, in other moments the same service can be a requestor or even an intermediate between a provider and a requester. That's the reason the web services are not client/server model.
Protocol Stack
- Service Transport: transport messages between applications (HTTP, SMTP, FTP)
- XML messages: encoding messages (SOAP)
- Service Description: describing the public interface to a web service (WSDL)
- Service Discovery: centralizing services into a common registry and providing easy publish/find functionality (UDDI)
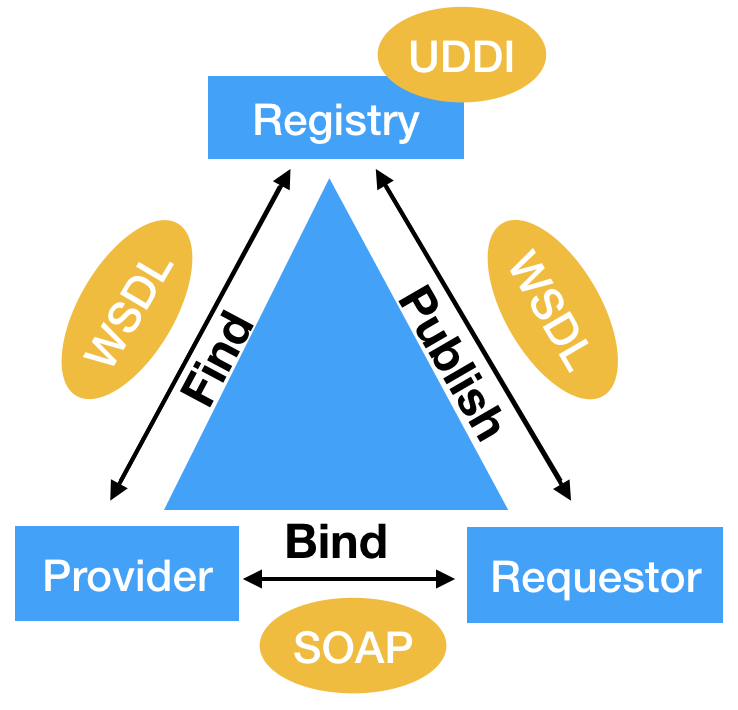
The WSDL usually has a service definition that includes the abstract definition (interface) and the concrete definition (implementation). The concrete definition is represented by binding, service and endpoint (port).
Communication
When we are talking about synchronized communication, the kind of message exchange is request/response. The asynchronous communication usually uses publish and subscribe or fire and forget.
The Correlation technic is used to match messages exchanged by different paths. In this case, is necessary to store the ID's.
Annotations
You can see detail about the annotations used to create a web service here. And below, some of them.
- @WebService: used in the class or interface that represent the web service. Some parameters are (a) name is mapped to the wsdl:portType from wsdl file, (b) the serviceName is mapped to wsdl:service, (c)the endpointInterface will be used only if you create a interface file.
- @WebMethod: it makes the operation in web service available. It has operationName, mapped to wsdl:operation and action.
- @SOAPBinding: "Specifies the mapping of the Web Service onto the SOAP message protocol".
- @HandlerChain: "Associates a Web Service with an external file that contains the configuration of a handler chain". The parameters are file and name.
- @WebResult: "Customizes the mapping between the Web Service operation return value and the corresponding element of the generated WSDL file". The name is mapped to wsdl:part.
- @WebParam: "Customizes the mapping between operation input parameters of the Web Service and elements of the generated WSDL". The name is mapped to wsdl:part.
Example
One example you can see on the Java Tutorial. This example will show you a class named Hello. The annotation @WebService define the endpoint class. The @WebMethod annotation defines what will be available to use.
importjavax.jws.WebService;
importjavax.jws.WebMethod;
@WebService
public class Hello {
private String message = new String("Hello, ");
@WebMethod
public String sayHello(String name) {
return message + name + ".";
}
}
To run this example you need to have a server (I used JBoss 7.0). Start your server and create a new Web Server. After that, select the class that you created. The next step is to create the wsdl document. Et voilà. The IDE creates all the structure for you.
![]() |
![]() |
![]() |
![]() |
To verify if it's working, get the address created on your wsdl (wsdlsoap:address) and put on your browser. The second test is to see the wsdl file by the browser. Use similar link but change to wsdl folder.
In my case, the addresses are:
- http://localhost:8080/WebServiceProject/services/Hello
- http://localhost:8080/WebServiceProject/wsdl/Hello.wsdl
![]() |
![]() |
Now you can test using a client class. Create the class, similar to the Java Tutorial example, then run such a Java Application. The result will be shown on the console.
import javax.xml.ws.WebServiceRef;
public class HelloClient {
@WebServiceRef (wsdlLocation =
"META-INF/wsdl/localhost_8080/WebServiceProject/Hello.wsdl"
private static Hello service;
public static void main(String[] args) {
System.out.println(sayHello("Malta");
}
private static String sayHello(java.lang.String param) {
Hello port = new Hello();
return port.sayHello(param);
}
}
Also, the web service can be tested using the SoapUI tool. In this case, you will create a test case and run. It will create an XML to request and result in an XML response.
The project is on my GitHub.
Interview Questions
A good way to get concepts objectively is by questions. Here are some good lists of questions that you should read.
- Web Services Interview Questions – SOAP, RESTful
- Top 17 SOAP Web Services Interview Questions & Answers
- soap Interview Questions and Answers
- Top 45 Web Services Interview Questions and Answers (RESTful, SOAP, Security questions)
Conclusion
Web service is a very significant subject. SOAP and REST have differences and you need to understand these to make a good choice.
This post is just the beginning to us. Go deeply and good luck.