Spring Data
The Spring Data is part of the Spring Ecosystem that is responsible for the persistence layer. The Spring Data has many projects within it where the best known is the Spring Data JPA.
It makes it easy to use data access technologies, relational and non-relational databases, map-reduce frameworks, and cloud-based data services. This is an umbrella project which contains many subprojects that are specific to a given database. [1]
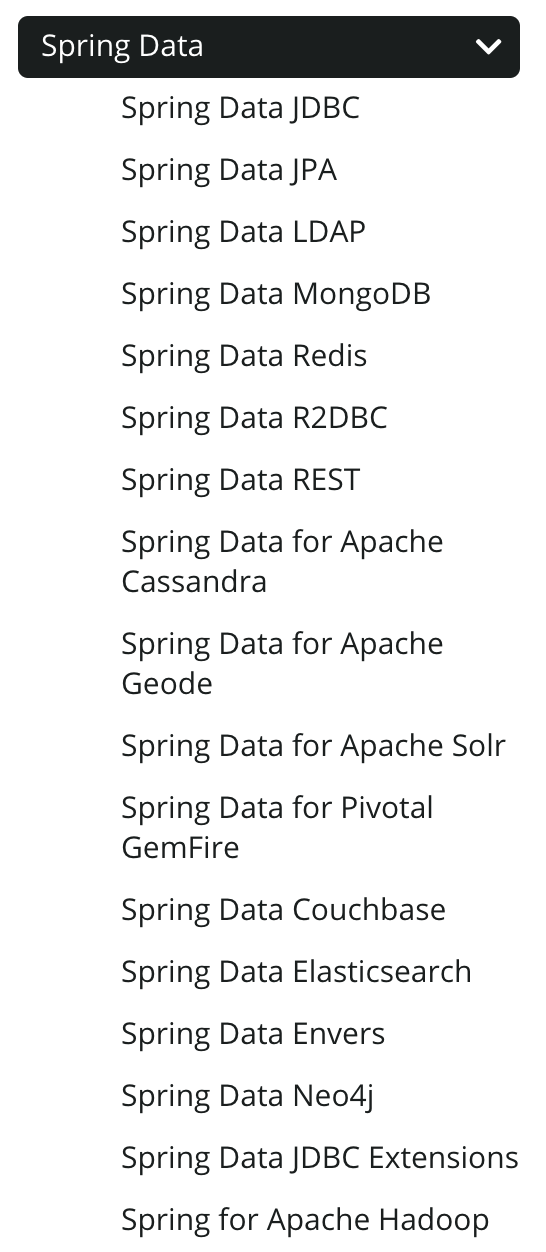
The Spring Data provides a common set of interfaces, a common naming convention, aspected behavior and repository and data mapping convention. The Repository interface (inspired by DDD) gives to developer the CRUD actions ready to use, avoid all the DAO implementations. Besides that, it also give support to sort results or to pagination. Then, the key components are Repository Interface and Entity Objects.
The example below show a repository with the method to consult student by name and you don't need to implement it. If you need a specific query you also can custom a query to it, eliminating the use of @NamedQuery on the entity classes.
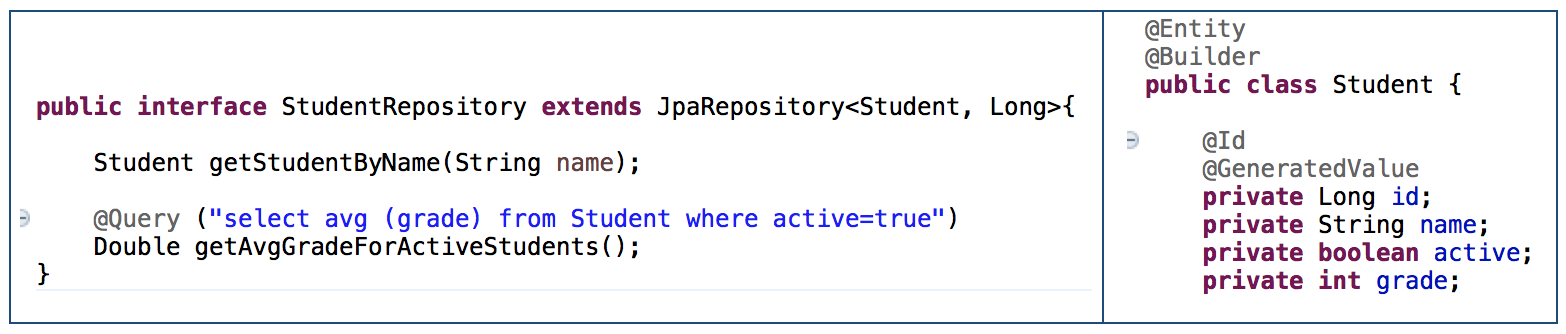
In summary, as the benefits, Spring Data remove boilerplate code and provide a clean mechanism to make swapping data sources easier.
Spring Data Commons
Core Spring concepts... provides shared infrastructure across the Spring Data projects. It contains technology neutral repository interfaces as well as a metadata model for persisting Java classes
- Powerful Repository and custom object-mapping abstractions
- Support for cross-store persistence
- Dynamic query generation from query method names
- Implementation domain base classes providing basic properties
- Support for transparent auditing (created, last changed)
- Possibility to integrate custom repository code
- Easy Spring integration with custom namespace
Maven
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-commons</artifactId>
<version>${version}</version>
</dependency>
Spring Data JDBC
Spring Data repository support for JDBC. In order to achieve this it does NOT offer caching, lazy loading, write behind or many other features of JPA. This makes Spring Data JDBC a simple, limited, opinionated ORM
If you need something simpler than JPA you can use JDBC. More detail about it you can see the documentation.
Maven
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-jdbc</artifactId>
<version>2.2.3</version>
</dependency>
Plus
Spring Data JPA
Spring Data repository support for JPA. Spring Data JPA aims to significantly improve the implementation of data access layers by reducing the effort to the amount that’s actually needed. As a developer you write your repository interfaces, including custom finder methods, and Spring will provide the implementation automatically
The way to code with JPA using Spring is similar how you code using JPA with Java EE. However, in Java EE , some code is too verbose. More detail about it you can see the documentation.
Maven
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-jpa</artifactId>
<version>2.4.0</version>
</dependency>
Plus
JPA | JPA e Hibernate |
---|---|
Spring Data LDAP
It provides repository abstractions for Spring LDAP on top of Spring LDAP’s LdapTemplate and Object-Directory Mapping
More detail about how to use LDAP Repositories you can see the documentation.
Maven
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-bom</artifactId>
<version>2021.0.3</version>
<scope>import</scope>
<type>pom</type>
</dependency>
Plus
Spring Data KeyValue
Map based repositories and APIs to easily build a Spring Data module for key-value stores.
More detail about it you can see the documentation.
Maven
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-keyvalue</artifactId>
<version>${version}.RELEASE</version>
</dependency>
Spring Data REST
Exports Spring Data repositories as hypermedia-driven RESTful resources in a simplest way then Spring MVC or Spring WebFlux.
More detail about how to use LDAP Repositories you can see the documentation.
Maven
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-rest-webmvc</artifactId>
<version>3.5.3</version>
</dependency>
Plus
Conclusion
This post had no intention to go deep into the Spring Data but to show Spring Data is more than JPA. If you need one of the Spring Data projects, go to the documentation and improve your knowledge.