Angular - Start
Introduction
Angular is an open-source JavaScript framework for building web applications and apps in JavaScript, HTML, and Typescript which is a superset of JavaScript. [1]
AngularJS is the first version of this framework. The highlight on that version to compare with following versions is AngularJS use JavaScript and Controller. From the second version, the Angular is completely different, which makes these versions not compatible. The new versions of Angular use TypeScript, Components, mobile compliant, lazy loading, use dependency injection and have CLI to support the projects (e.g: create project, create components).
Now, Angular is owned by Google that is promising to deliver a new version every 6 months.
Pay attention: even Angular having TypeScript as language, Angular transpile/convert it to JavaScript, the language the browser really know.
The three parts of the Angular are:
- Angular Core: central part of the angular
- Angular CLI: Start new projects
- Angular Materials: UI Component Library
Architecture
The parts of the framework to know are:
- Template: the HTML view of Angular to write directives. It can be create inline or creating a separate HTML file
- Component: Binds the View and Model
- Module: Groups components logically
- Binding: define how view and component communicate (expression inside the HTML tags or property biding)
- Directives: binding values and changes the HTML DOM behaviors. It can be Structural, Attribute or Component.
- Services: helps to share common logic across the project
- DI: pattern to create instance within the components across constructor. To make ir works should be used the providers attributes in metadata. It helps decouple class dependency.
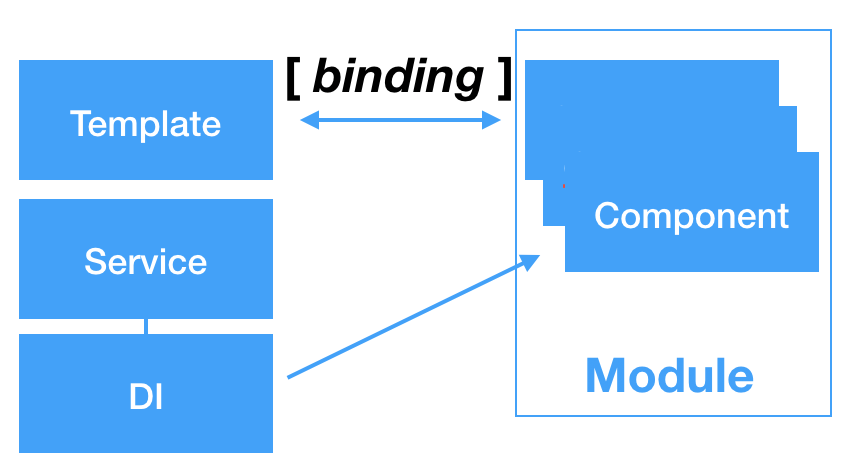
The resources around the Angular to support the developments are:
- SPA (Single Page Application): where the UI is loaded once and load parts by demand.
- Routing: collection of URL and correspondent component. Define navigation
- Lazy Loading: load by demand
- Pipe: transform a data to another format.
Start
To start to work with Angular you need to have:
- Nodejs
- Download and install node.js (https://nodejs.org/en/)
- Npm
- It will be installed with nodejs.
- Angular CLI
- IDE for writing your code
- Visual Studio Code (you can use other options)
The line commands you can need is below. If the CLI is old you should uninstall that, clean the cache and install another one.
$ [sudo] npm install -g npm
$ sudo npm uninstall -g angular-cli @angular/cli
$ npm cache clean --force
$ [sudo] npm install -g @angular/cli
// If you are using yarn
$ yarn global add @angular/cli
$ ng set --global packageManager=yarn
After that, you are ready to create your first project.
ng new my-project
Now, go inside the project you created and start the server.
cd my-project ng serve Test: http://localhost:4200/.
Here is the basic structure that the angular will create for you.
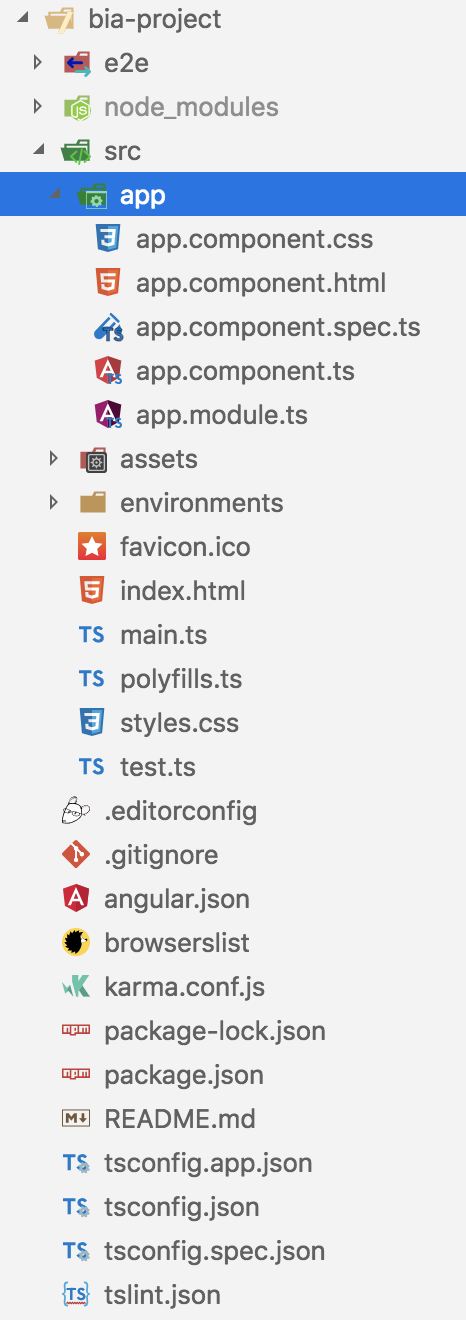
Most of the files are related to configurations and we don't have to be worried about that. The file angular.json, for example, has the dependencies and the node_modules has the real dependencies.
The src folder has the main source code that we will work. The app folder has the file that we will work to show in the browser. For every updates, you just need salve your file and the server will be updated and you can see the result in the browser.
For a test, go to the app.component.html and change the title. This file also has a variable defined in the app.component.ts. Change this as well and see the result. The app.component.ts is the typescript file that defines the component.
The same app.component.ts file has a "selector" that define "app-root". You will see that in the index.html page. That is all the magic.
For more detail about what does mean each file in the project can see that in here.
A simple modification - Module
A simple test that you can do just is adding a new module. The module is a set of functional blocks related to an application domain. The module created by default is the @NgModule. Angular is defined by a set of NgModules, which is a set of functional blocks related to an application domain. It declares a compilation text for a set of components. The NgModule is identified by @NgModule() decorator.
Decorator is a Metadata/Annotation to inform to Angular what kind of class it is ( @Component, @NgModule )
One example is to add a dynamic input text. To do this, delete all the content of the app.component.html and put this:
< input type = "text" [(ngModel)]= "title" >
< p > { { title } } < /p >
Now, go to the app.module.ts and import the new module and add the module in the imports array.
Save all files and the result is, what you write in the field it will be shown below.
Other examples you can find here.
Adding bootstrap
To use bootstrap you need to install that. If you want a specific version you just need to add that on the end of the command.
npm install --save bootstrap@3
Refresh your project and the folder will be shown inside the node_modules folder. Now, update the file Angular.json adding in styles tag "node_modules/bootstrap/dist/css/bootstrap.min.css".
PS: As the studies progress, I will add the code in the github project.
Commands++
- npm: package manger
- "ng serve" builds in memory while "ng build" builds on the hard disk
- "ng Build --prod" compress and reduces files
References
- https://github.com/angular/angular-cli/wiki
- https://www.tutorialspoint.com/angular7/angular7_quick_guide.htm
- https://www.udemy.com/the-complete-guide-to-angular-2/
- https://blog.angular-university.io/getting-started-with-angular-setup-a-development-environment-with-yarn-the-angular-cli-setup-an-ide/